Hello!
Welcome to my blog for my youtube channel Stick2Tech!
Today I will start with JavaScript Fundaments and go little deeper on classes, inheritance etc.
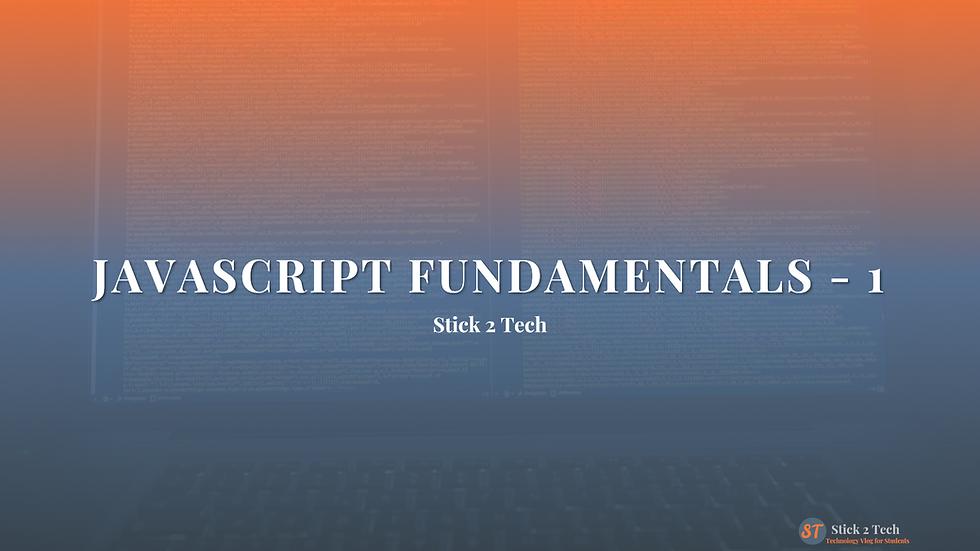
What is JavaScript?
“JavaScript is a cross-platform, object-oriented scripting language used to make webpages interactive”.[1]
“Inside a host environment (for example, a web browser), JavaScript can be connected to the objects of its environment to provide programmatic control over them.”[1]
JavaScript has standard core libraries for example Array, Math, Date etc. There are many other libraries and framework developed on these base libraries.
Is JavaScript and Java the same?
No, they are not the same.
JavaScript Syntax resembles Java so it is similar but have many differences
JavaScript is free form because you do not have to define type of variable.
JavaScript does not have access to hard drive to write.
There are more differences that can be found the Mozilla Site.
Advantages of using JavaScript?
In JavaScript there are various libraries and frameworks available for speedy development of code. While choosing these frameworks and libraries you have know what type of functionalities you are looking for from them.
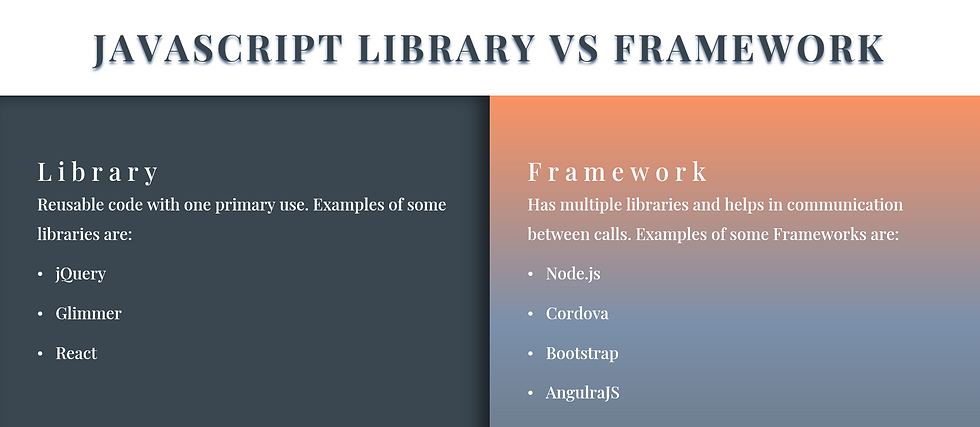
While JavaScript is mainly used in the Client side (mainly in browsers) there are libraries and framework for the Server side too.
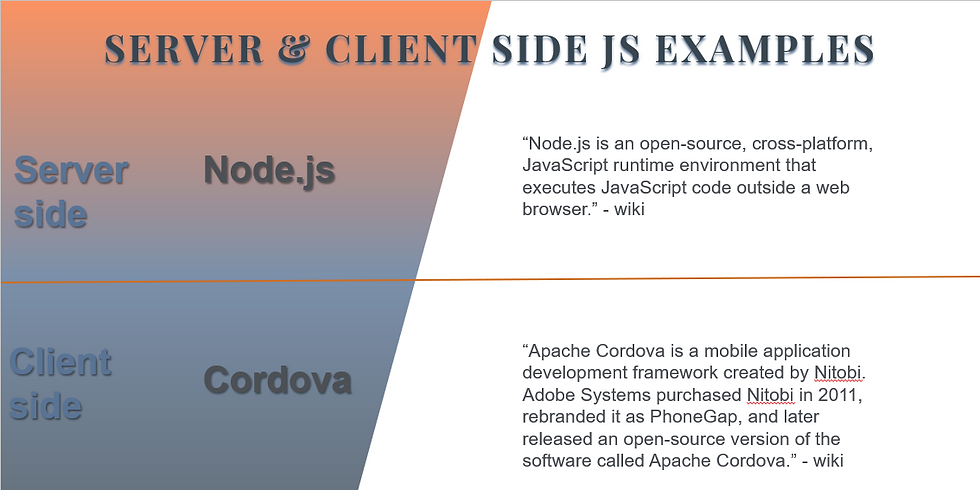
1. Basic concepts
a. Constant and variable
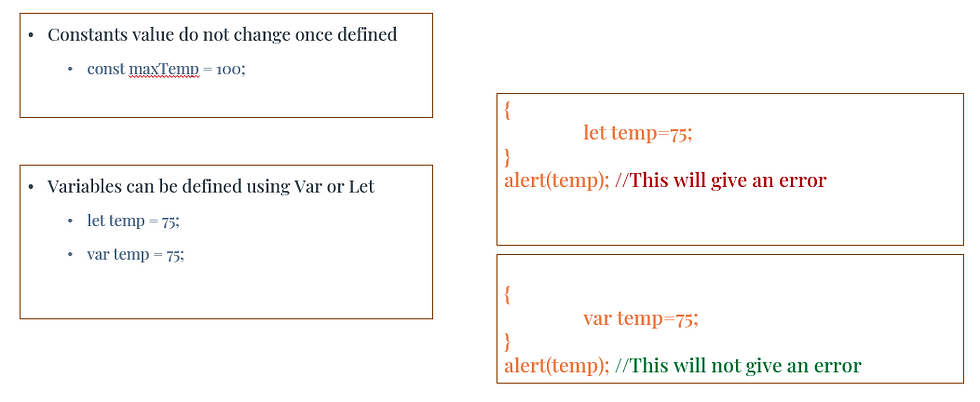
Constant can be defined with const key word whereas variables can use either var or let keyword. let has scope within {} brackets where as var does not have scope. So, you have to choose variable type to your need.
b. Array
Array can hold one or more variable(s)
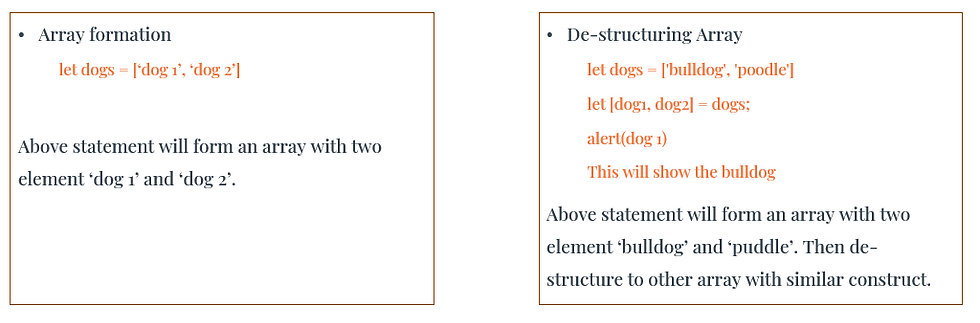
Arrays can be de-structured to another array of similar structure by assigning the arrays.
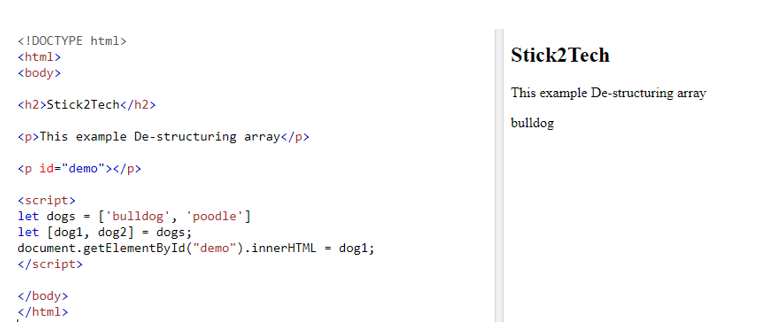
The above example shows code that de-structures array and is used to display in a html page.
c. Primitive Data types
The following primitive data types are available in JavaScript.
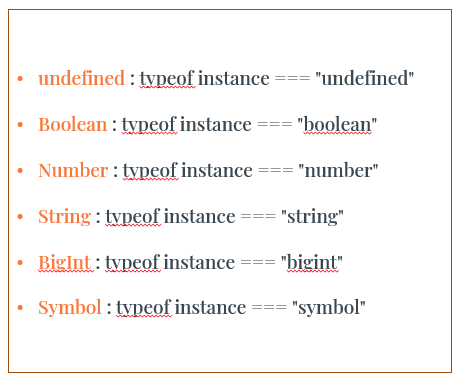
d. TypeOf() and Type conversion
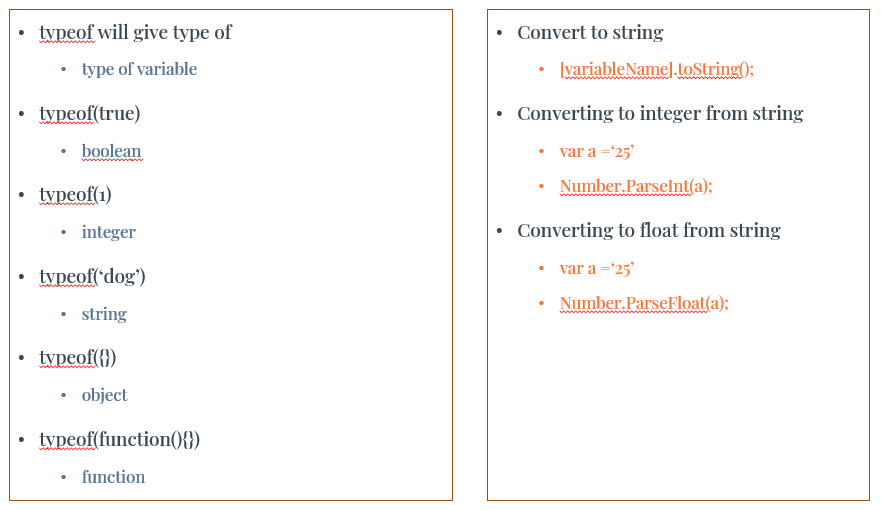
e. Equality and Relational Operators
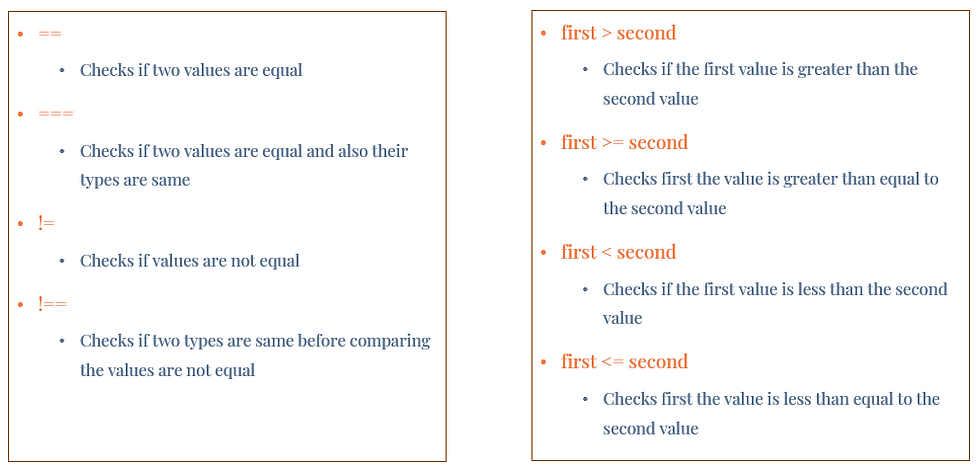
f. Unary and Boolean Operators
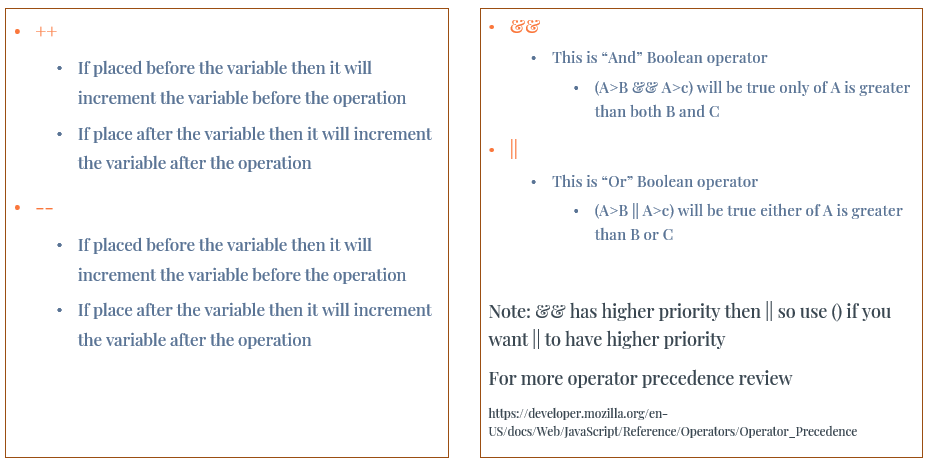
More details can be found in this article from Mozilla.
g. Loops and control in JavaScript
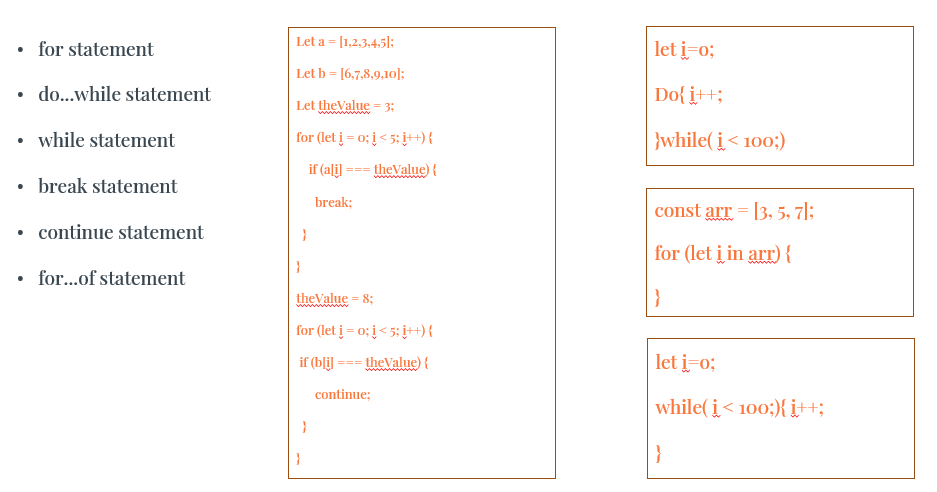
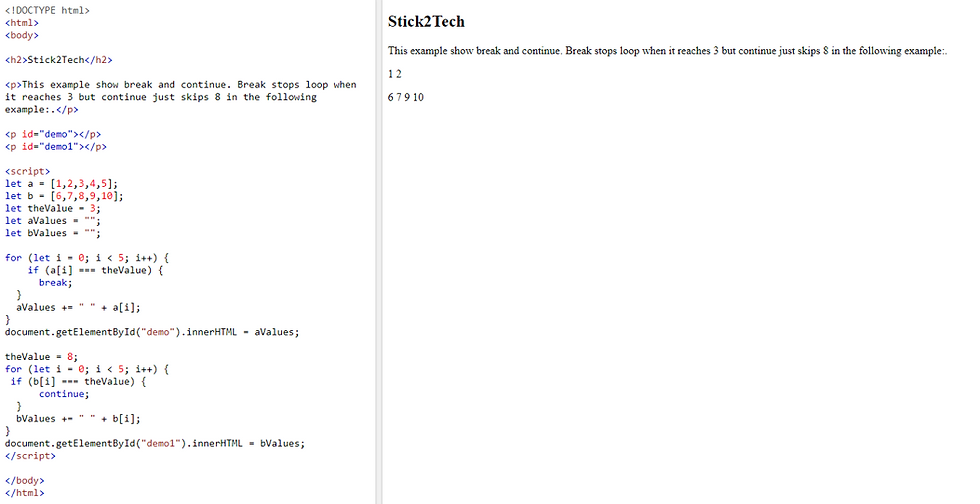
2. Functions, Objects and Classes concept
a. Basics of Functions, Objects and Classes
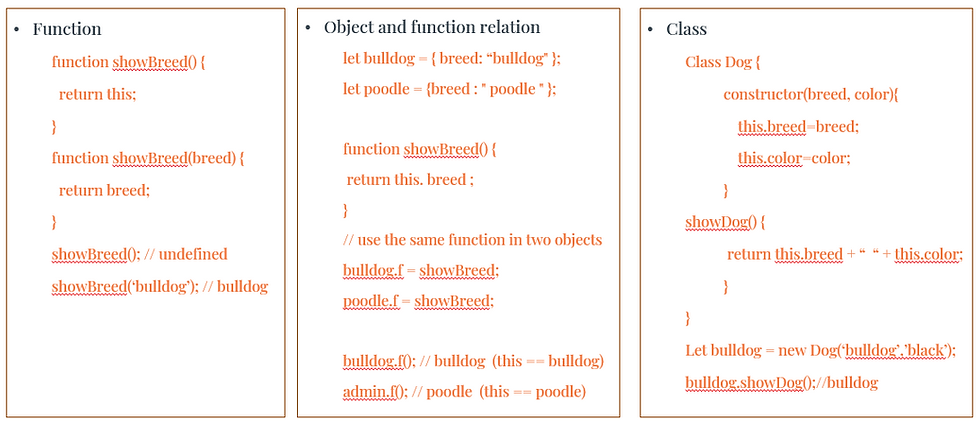
First example is a function where the "this" keyword is undefined.
Second example is function used along with JavaScript Object where this represents the current object that function is working with. Here the "this" keyword is the object that function is working with.
The third example uses class which is building blocks of object oriented programming. The "this" keyword in the class is used for the current instantiated object which is bulldog.
b. Parameter passing in functions
Parameters can be passed as regular parameters as in example 1 below, or using rest Parameters with a ... as in example2 below or with default value as in example 3 below.
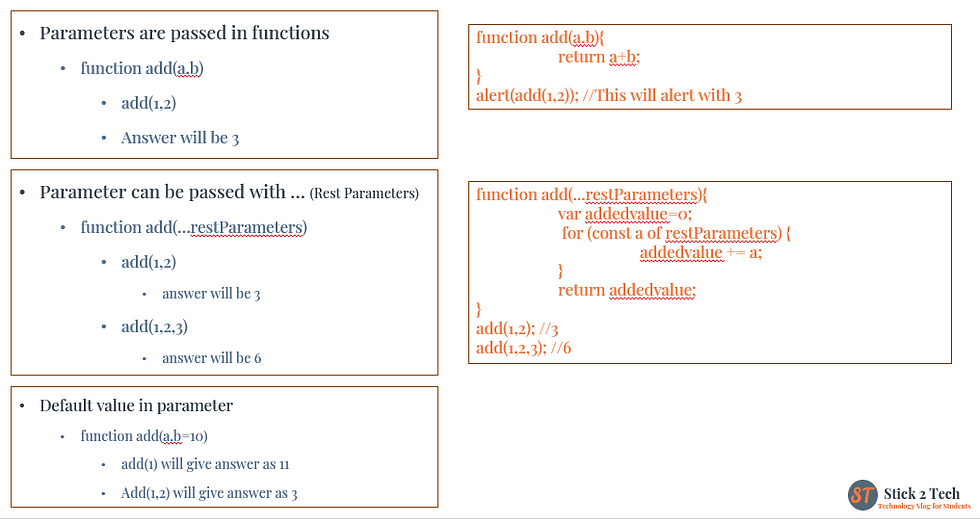
Following example shows example of parameter passing along with rest parameters.
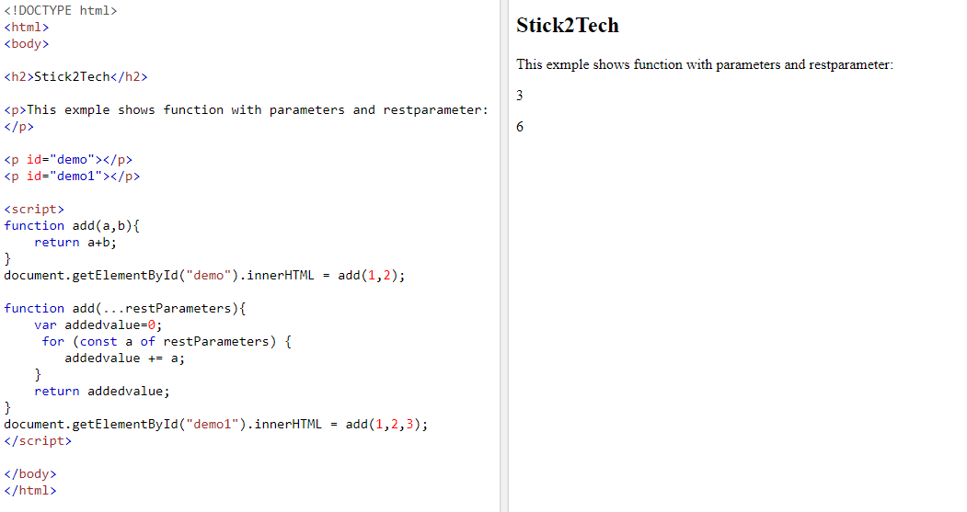
c. Arrow functions
Arrow functions use arrows to indicate the input parameters and return values(anything following the arrow is the return value, the value(s) before is/are the parameters)
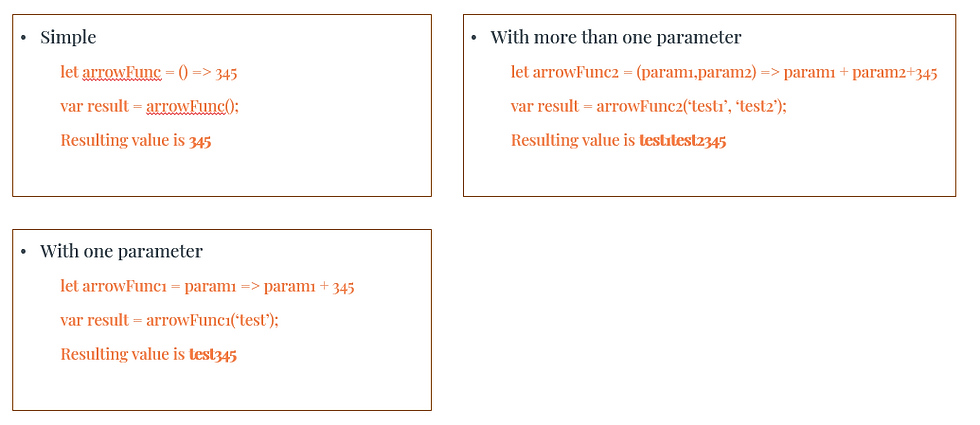
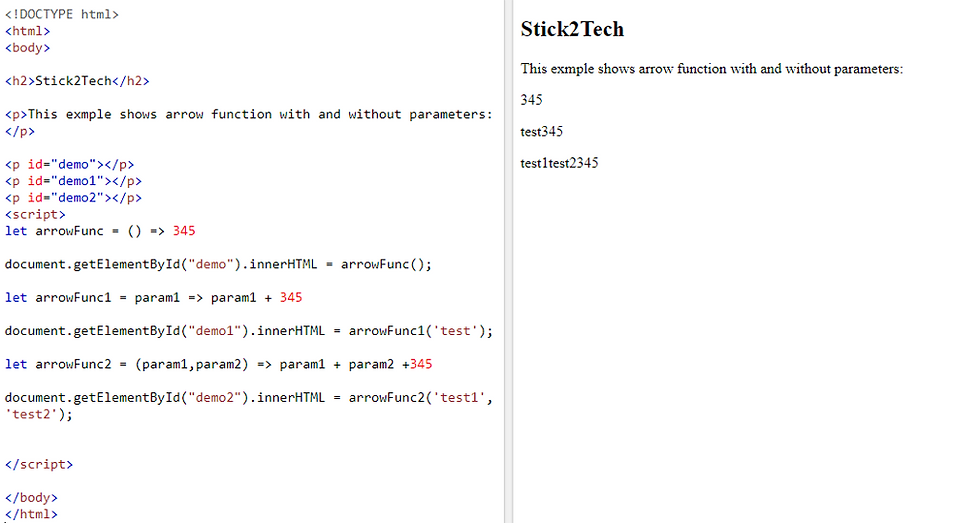
d. Functions and IIFE (Immediately Invoked Function Expression)
IIFE, as the name suggests, executes the function immediately after creation and puts the value in result which can be used later-on.
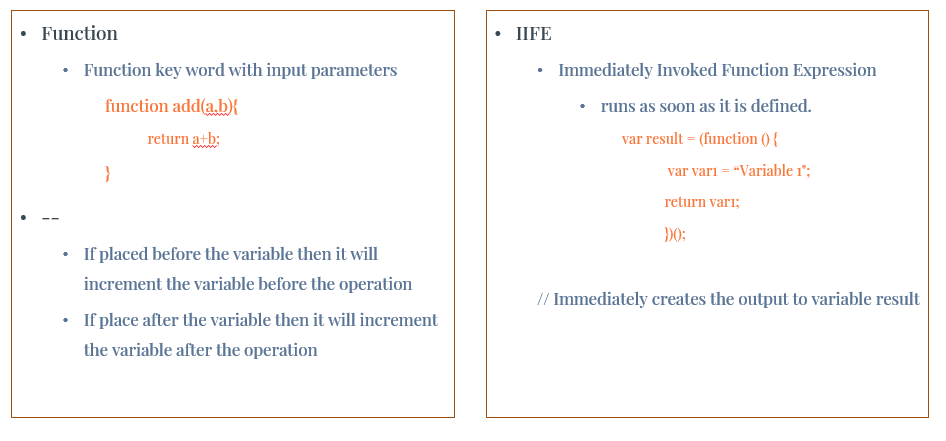
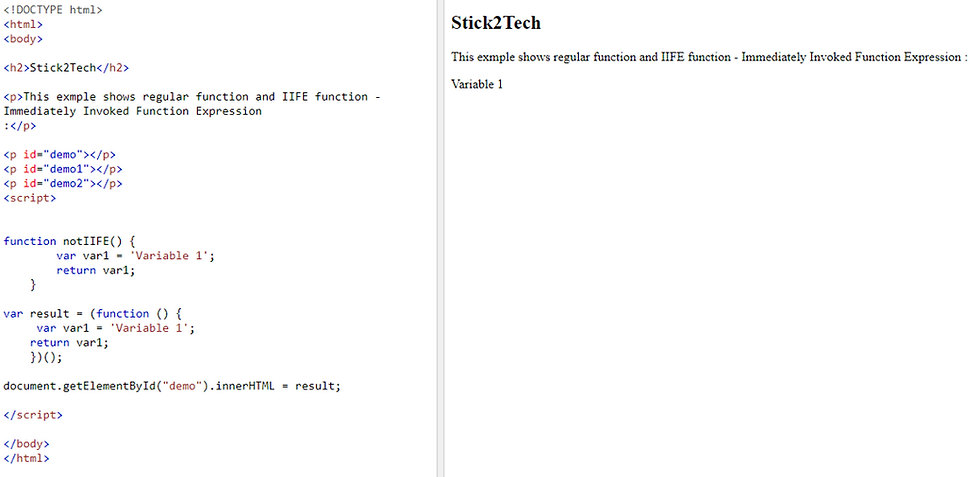
e. Lexical scope and closure function
Lexical scoping shows that the variable within the parent function is available in the enclosed function.
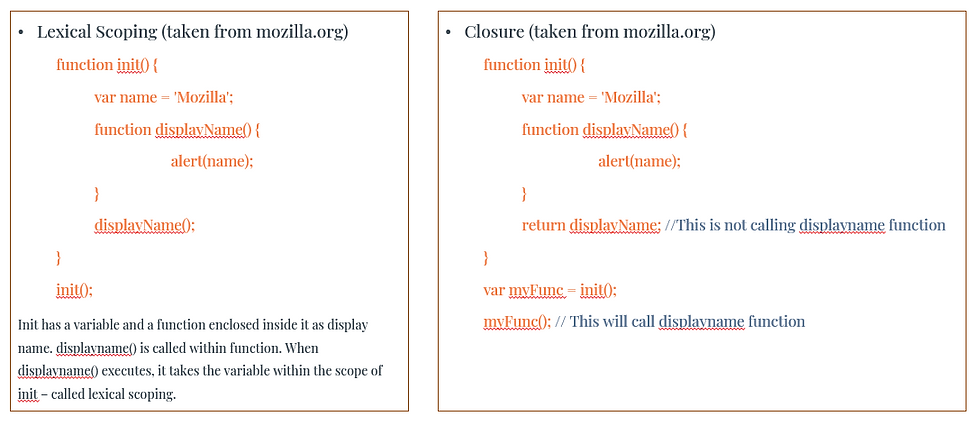
Following code shows that the variable within the functions can be used only within the enclosed method.
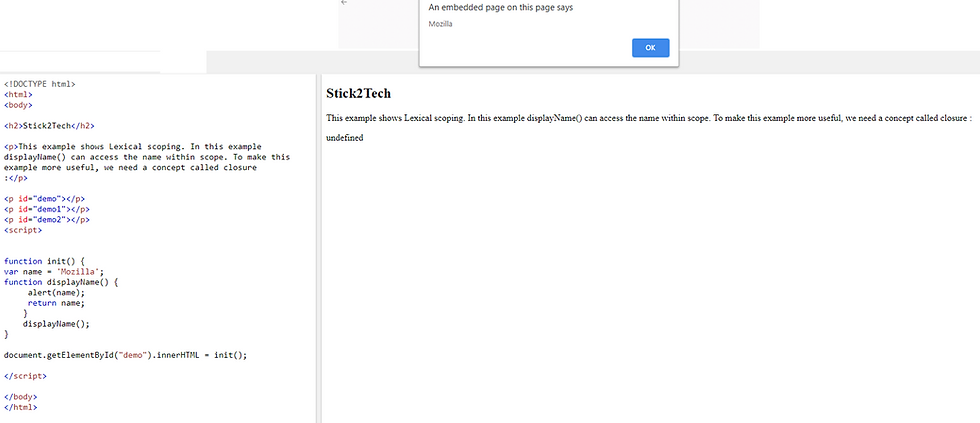
To expose the inner method out, we need a concept called closure where inner method's reference can be assigned to a variable that can be exposed outside the function.
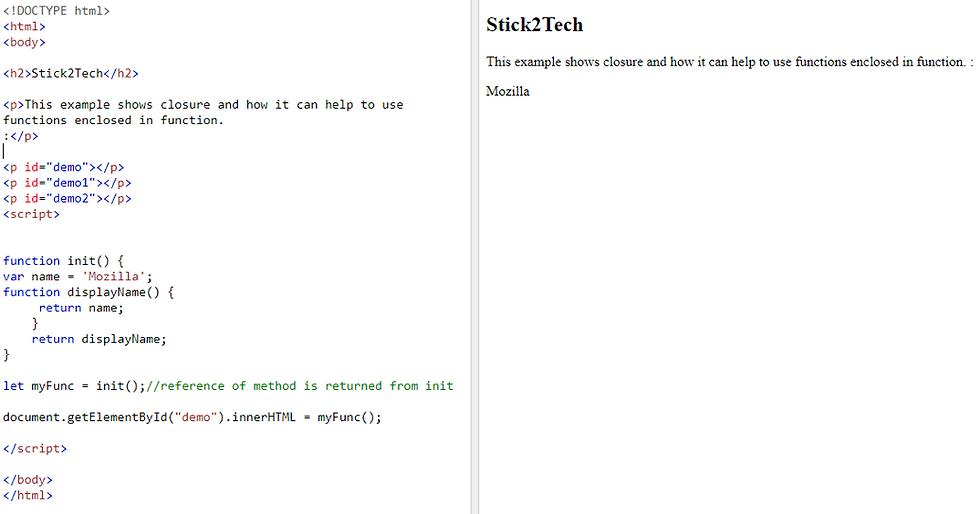
3. Function, Objects and Classes intermediate concepts
a. JSON (JavaScript Object Notation)
Object is JavaScript which is also called JSON can be used to store data and communicate object information in name:value pair as shows below. The object can also be de-structured to other similar object.
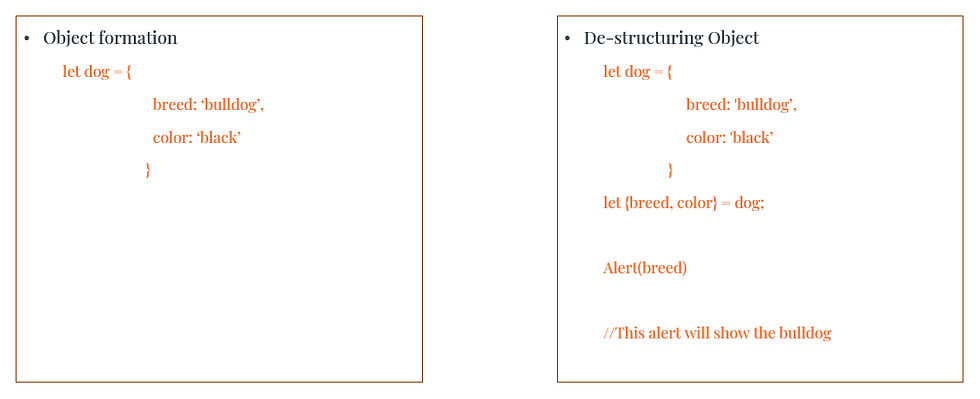
Following example show how the object can be de-structured and used in an web-page.
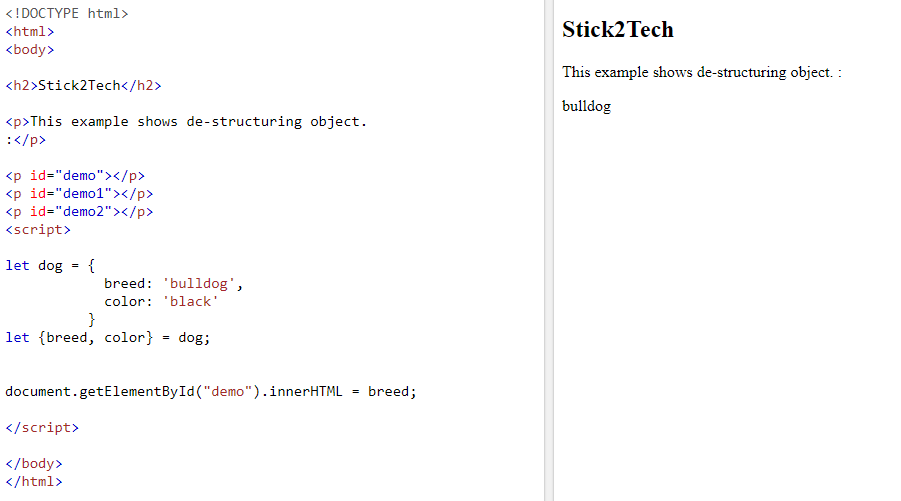
b. Getter Setter in an Object
Getter will help to get a property of an object and setter will help to set property of an object
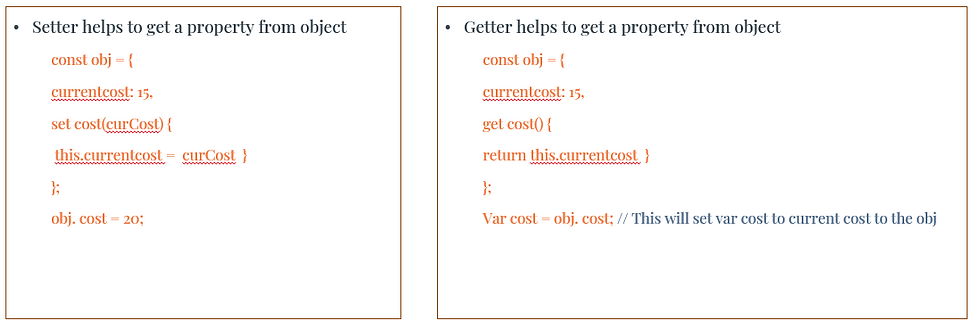
In the following example, we get the current cost using getter and then used setter to set the cost and used the getter to get the current cost to make sure setter updated the cost to the new value.
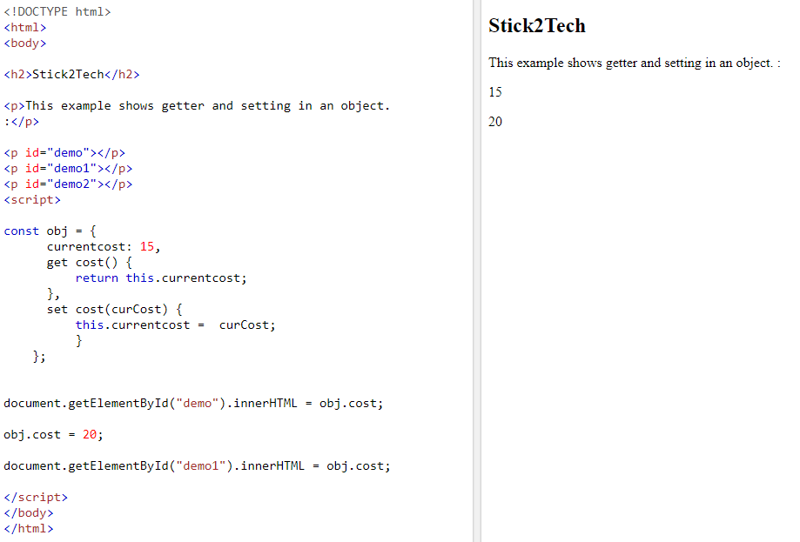
c. Bind, call, apply in functions
Bind is used to create a new function based on a function. This can also be viewed as an instance of the function that works similar to an object. Call is similar to binding but it will call the function immediately. Apply is similar to call but it can be used to pass arguments.
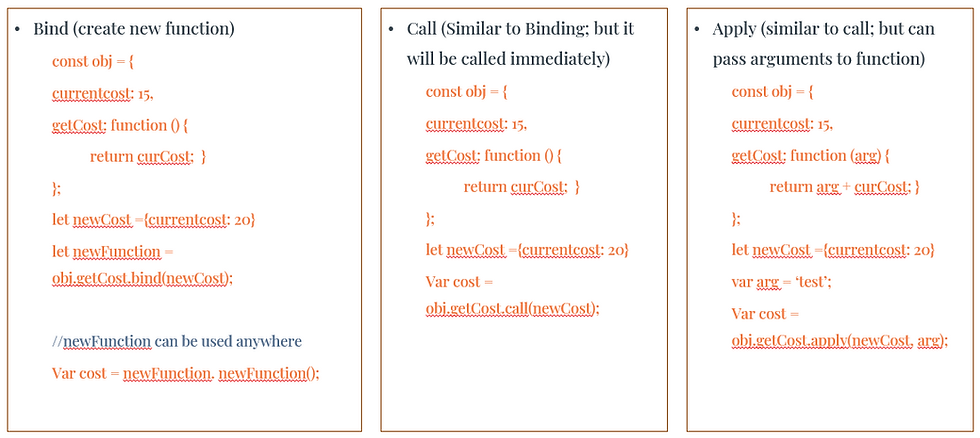
d. Classes and objects
Classes can be formed using functions or using classes as shows in two examples below. Objects can be instantiated based on the classes using the "new" keyword.
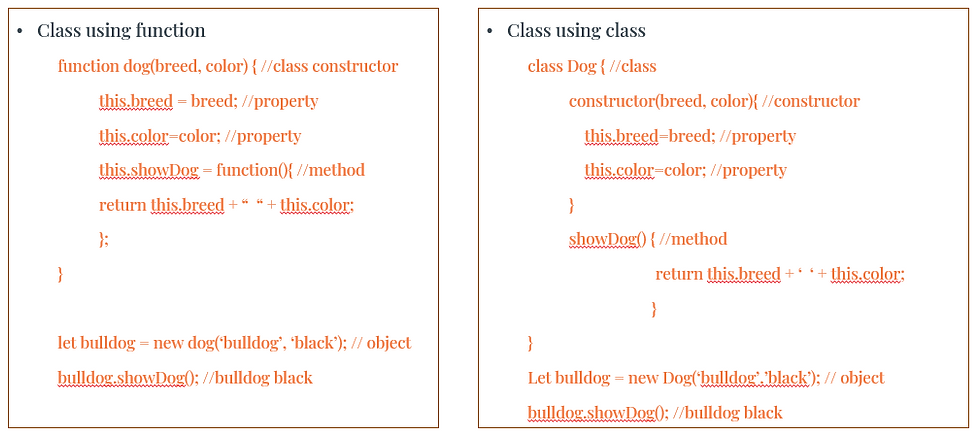
Following is an example of a class using function:
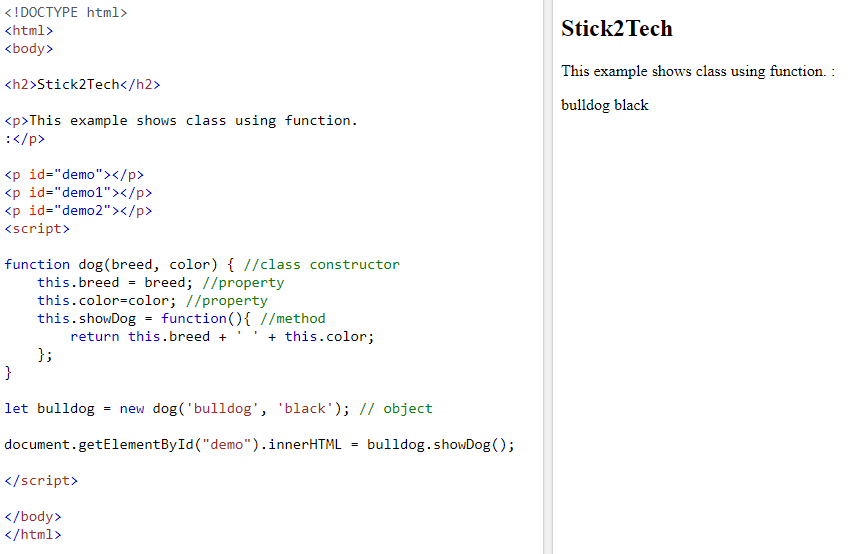
Following is an example of a class using Class:
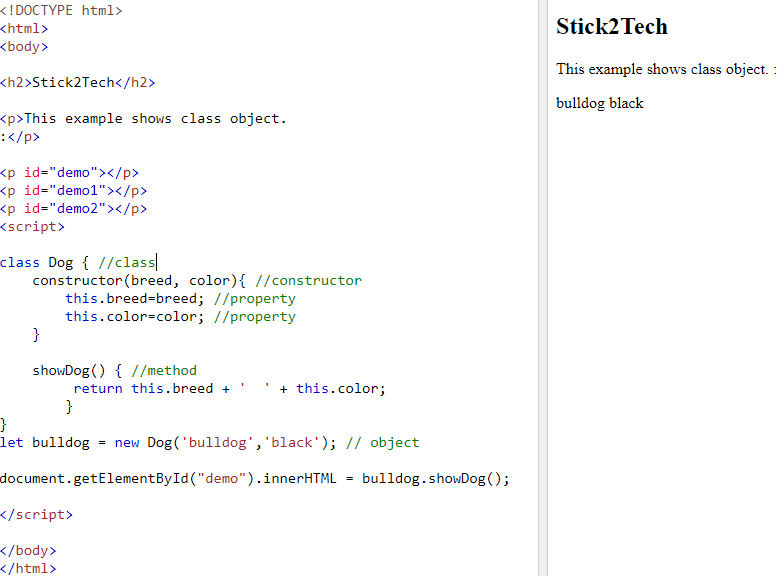
e. Inheritance
Inherence helps the create parent classes where child can inherit some properties and methods.
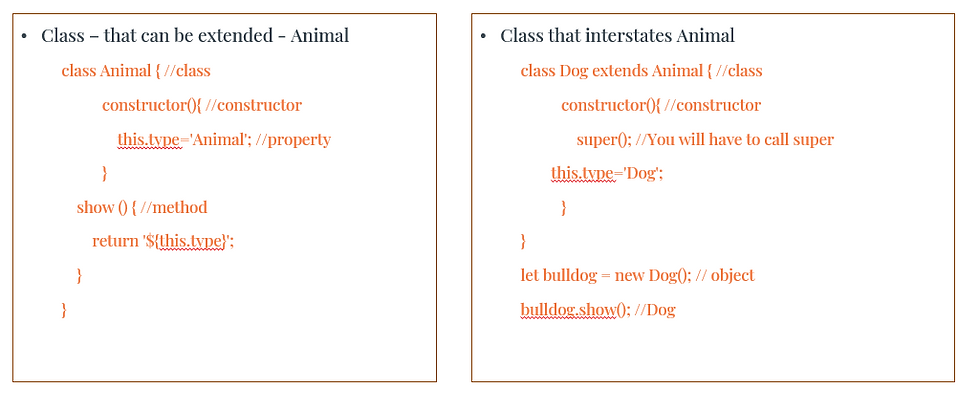
Following is the code sections showing how inheritance can be used.
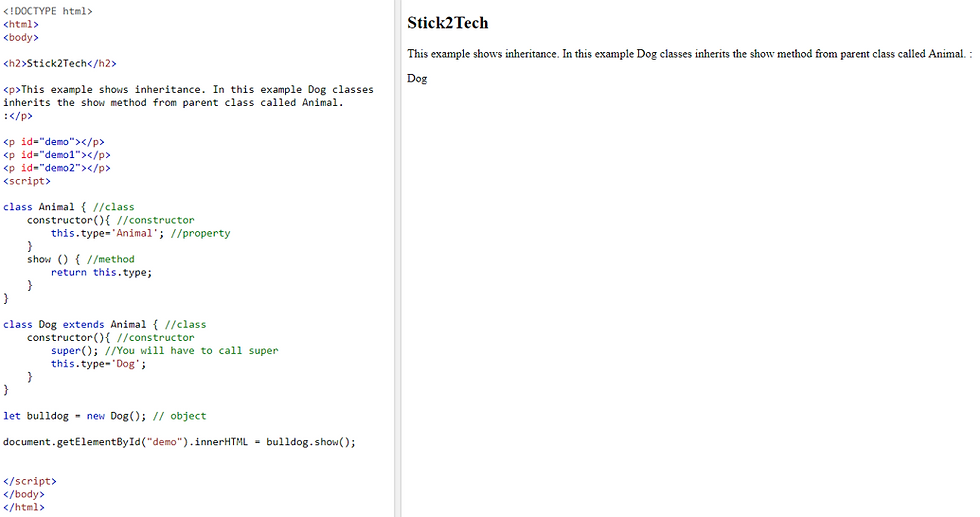
f. Module export and import
We can create module based on any class by using an export keyword and can be imported in one to many places where the module is needed by using an import keyword.

Thank you for reading my Blog about JavaScript, I will be making other blogs relatively soon and I want to hear your opinion about what I should talk about? Should I go more in-depth with this topic, or is there something you want specifically like programming in relation to JavaScript, if this topic interested you, I encourage you to do your own research on the subject, or write in the comments below if you have any questions for me.
Acknowledgments:
[1] https://developer.mozilla.org/en-US/docs/Glossary/IIFE
Comments